String: In C programming, a string is a sequence of characters terminated with a null character \0.
Example:
char c[] = “bangladesh”;
How to declare a string?
Here’s how you can declare strings:
char s[5];
How to initialize strings?
You can initialize strings in a number of ways.
char c[] = “abcd”;
char c[50] = “abcd”;
char c[] = {‘a’, ‘b’, ‘c’, ‘d’, ‘\0’};
char c[5] = {‘a’, ‘b’, ‘c’, ‘d’, ‘\0’};
Assigning Values to Strings
Arrays and strings are second-class citizens in C; they do not support the assignment operator once it is declared. For example,
char c[100];
Example 1: scanf() to read a string
#include
int main()
{
char name[20];
printf(“Enter name: “);
scanf(“%s”, name);
printf(“Your name is %s.”, name);
return 0;
}
Output
Enter name: Dennis Ritchie
Your name is Dennis.
strcmp
#include "stdio.h" #include "string.h" int main() { char word_1[1000], word_2[1000]; printf("Enter 1st string: "); gets(word_1); printf("Enter 2nd string: "); gets(word_2); if (strcmp (word_1, word_2) == 0) printf ("Strings are same. \n"); else printf ("Strings are not same. \n"); return 0; }
strcpy ()
#include "stdio.h" #include "string.h" int main() { char word_1[1000], word_2[1000]; printf("Enter 1st string: "); gets(word_1); strcpy (word_2, word_1); puts (word_2); return 0; }
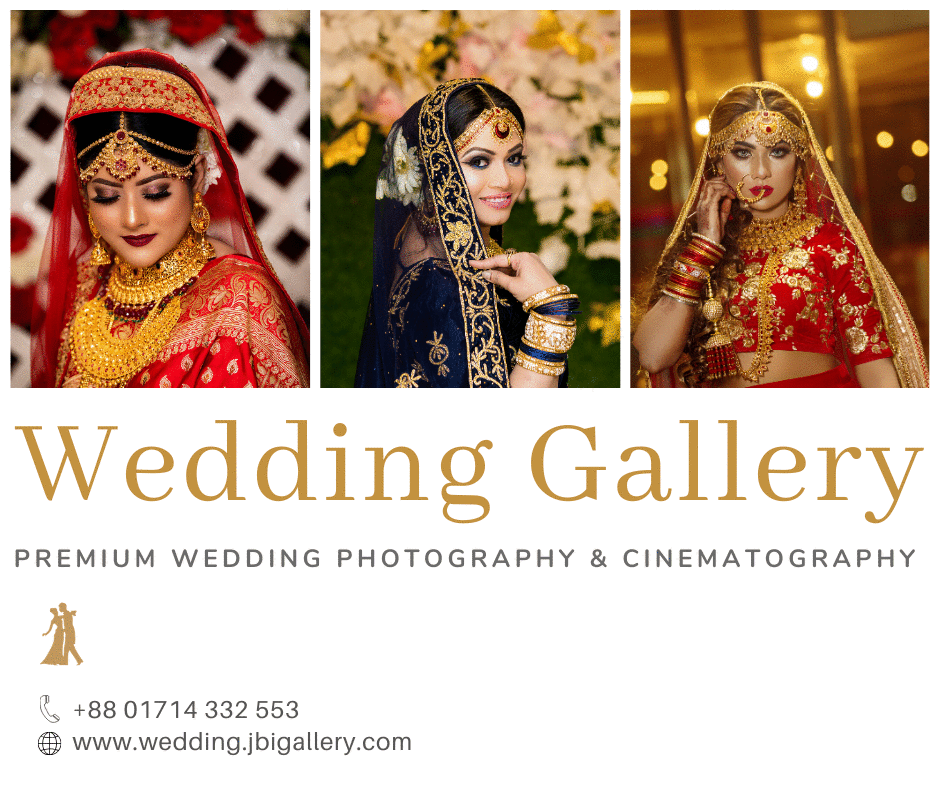